Why SOLID and Clean Code Principles in .NET Core Are Still Your Best Friends
- Maryanne
- 6 days ago
- 4 min read
Let’s face it: a lot of us geeks love to run after shiny new frameworks or fancy libraries. .NET 8 C# 13! Minimal APIs! But do you know what’s even cooler? Writing code that doesn’t leave future you banging your head against a wall. Yes, I’m talking about SOLID principles and clean code. They’ve been around for a while, but trust me, they’re not about to go the way of VHS tapes. They’re more like vinyl records—a classic that just keeps coming back into style.
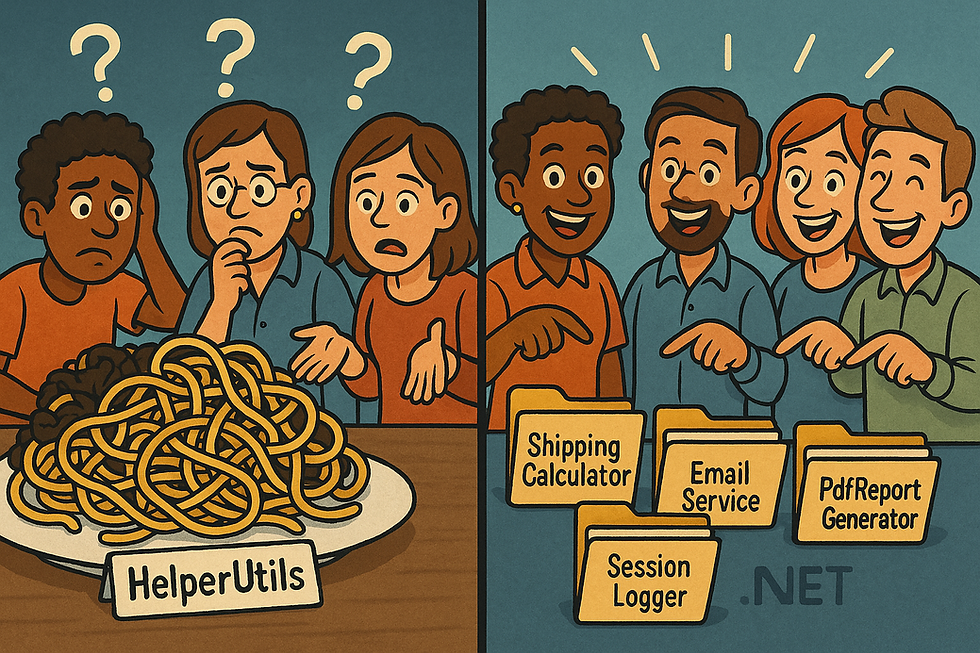
A Quick Refresher: What’s SOLID Anyway?
SOLID is an acronym that stands for:
S ingle Responsibility Principle (SRP)
O pen/Closed Principle (OCP)
L iskov Substitution Principle (LSP)
I nterface Segregation Principle (ISP)
D ependency Inversion Principle (DIP)
If you’re thinking, “Great, just another set of rules my code is probably violating,” don’t worry. We’ll dive into at least one of these principles in detail and hopefully spare you the tears later.
Why Should You Care?
You might be asking, “Isn’t .NET Core so advanced that it basically writes itself?” Sorry to burst your bubble, but no. (Though if you do find that AI tool, let me know. Maybe writing it's self is sooner then we think... ) In the meantime, here are a few reasons these timeless concepts still matter:
Code Maintainability Imagine you’ve just come back from vacation and open a your project that you built six months ago. If your code is SOLID, you’ll smile happily, make your changes quickly, and resume life as a coding rock star. If it’s not, you’ll probably spend the rest of your day unraveling method calls that look like a spaghetti-monster cameo in a horror film.
Scalability in Modern Architectures When your application grows, or you decide to go wild with microservices, clean, modular code helps you slice and dice functionality into smaller pieces without breaking everything else. No more feeling as if you’re performing a delicate surgery every time you want to add a new feature.
Faster Onboarding and Collaboration Let's not forget the real world: teams. You’re not (always) coding alone in a dark basement. A well-structured app using these principles lets new developers ramp up quickly. Less confusion, fewer frantic Slack messages. Win-win.
Single Responsibility Principle: The Star of the Show
Of all the SOLID principles, Single Responsibility Principle (SRP) is probably the most fundamental. It states:
“A class should have one and only one reason to change.”
In simpler terms, each class or service you write should do exactly one thing (and do it well). If you find yourself with a 1,000-line monstrosity that handles database operations, logging, email sending, and generating PDF reports, you probably need to break it down. For your sanity and the sanity of your teammates.
Why SRP Matters
Maintenance Bliss: If a class is responsible for just one behavior, you’ll know where to go when that behavior changes. Need to switch from SendGrid to Amazon SES for emails? Adjust the email service. No rummaging through a labyrinth of random lines in a single “mega-class.”
Testability: Smaller, single-purpose components are easier to test. You don’t have to mock half your entire application to confirm that a single method works.
Team Collaboration: Each developer can own a distinct part of the code, with fewer merge conflicts and fewer “Wait, you changed that line?” fiascos.
Picture a “HelperUtils” class—because of course that’s what we do when we don’t know where else to put something. This class calculates shipping costs, sends welcome emails, logs a user’s session info, and probably fetches coffee. Why not, right?
Before SRP: You have one colossal class that tries to do all the things and does them in 1,500 lines of tangled code. You open it, weep, then close your IDE because you can’t deal.
After SRP: You have ShippingCalculator, EmailService, and SessionLogger classes. Each focuses on its own job, and they don’t know or care about the intricacies of the others.
Bad Class Bad Class!
public class HelperUtils
{
public void CalculateShippingCost(Order order)
{
// Shipping cost logic.
Console.WriteLine("Shipping cost calculated!");
}
public void SendWelcomeEmail(User user)
{
// Email sending logic.
Console.WriteLine("Welcome email sent!");
}
public void LogSessionInfo(Session session)
{
// Logging logic.
Console.WriteLine("Session logged successfully!");
}
public void GeneratePdfReport(DataSet data)
{
// PDF generation logic.
Console.WriteLine("PDF generated!");
}
}
Good Classes, Very Good Classes
// A small model class for demonstration.
public class Order
{
public decimal TotalWeight { get; set; }
public decimal CostOfGoods { get; set; }
}
public class User
{
public string Name { get; set; } = "";
public string Email { get; set; } = "";
}
public class Session
{
public string SessionId { get; set; } = "";
public DateTime StartTime { get; set; }
}
public class ShippingCalculator
{
public decimal CalculateShippingCost(Order order)
{
// Example: base shipping + weight-based surcharge
decimal baseShipping = 5.00m;
decimal weightSurcharge = 0.75m * order.TotalWeight;
decimal totalShipping = baseShipping + weightSurcharge;
Console.WriteLine($"Shipping cost calculated: {totalShipping:C2}");
return totalShipping;
}
}
public interface IEmailSender
{
// Contract for sending an email
void SendEmail(string toAddress, string subject, string body);
}
public class SmtpEmailSender : IEmailSender
{
public void SendEmail(string toAddress, string subject, string body)
{
Console.WriteLine($"[SMTP] Email to {toAddress} - Subject: {subject}");
}
}
public class EmailService
{
private readonly IEmailSender _emailSender;
public EmailService(IEmailSender emailSender)
{
// Accept different email senders (SMTP, API, etc.)
_emailSender = emailSender;
}
public void SendWelcomeEmail(User user)
{
// Call your chosen email sender
_emailSender.SendEmail(user.Email, "Welcome!", $"Hey {user.Name}, thanks for joining!");
}
}
public class SessionLogger
{
public void LogSession(Session session)
{
// Could go to database, file, etc.
Console.WriteLine($"Session {session.SessionId} started at {session.StartTime}");
}
}
public class PdfReportGenerator
{
public void GenerateSalesReport(decimal totalSales)
{
// Stub logic; in reality, you'd use a PDF library
Console.WriteLine($"PDF generated for total sales: {totalSales:C2}");
}
}
Remember: SOLID principles and clean code are always in style. They’re your safety net when new requirements pop up, your codebase expands, or you need to integrate fancy new features—like an AI that picks the perfect cat meme to send your users (seriously, someone should build this). If you keep these core practices in mind, you can avoid monstrous classes and system-wide refactoring emergencies.
So go forth, wise coder, and banish your HelperUtils once and for all. Your future self—and your fellow developers—will thank you.
Commentaires